You don't need third-party libraries to read CSV file in Python! Python's csv
module includes helper functions for reading CSV files, tab-delimited files, and other delimited data files.
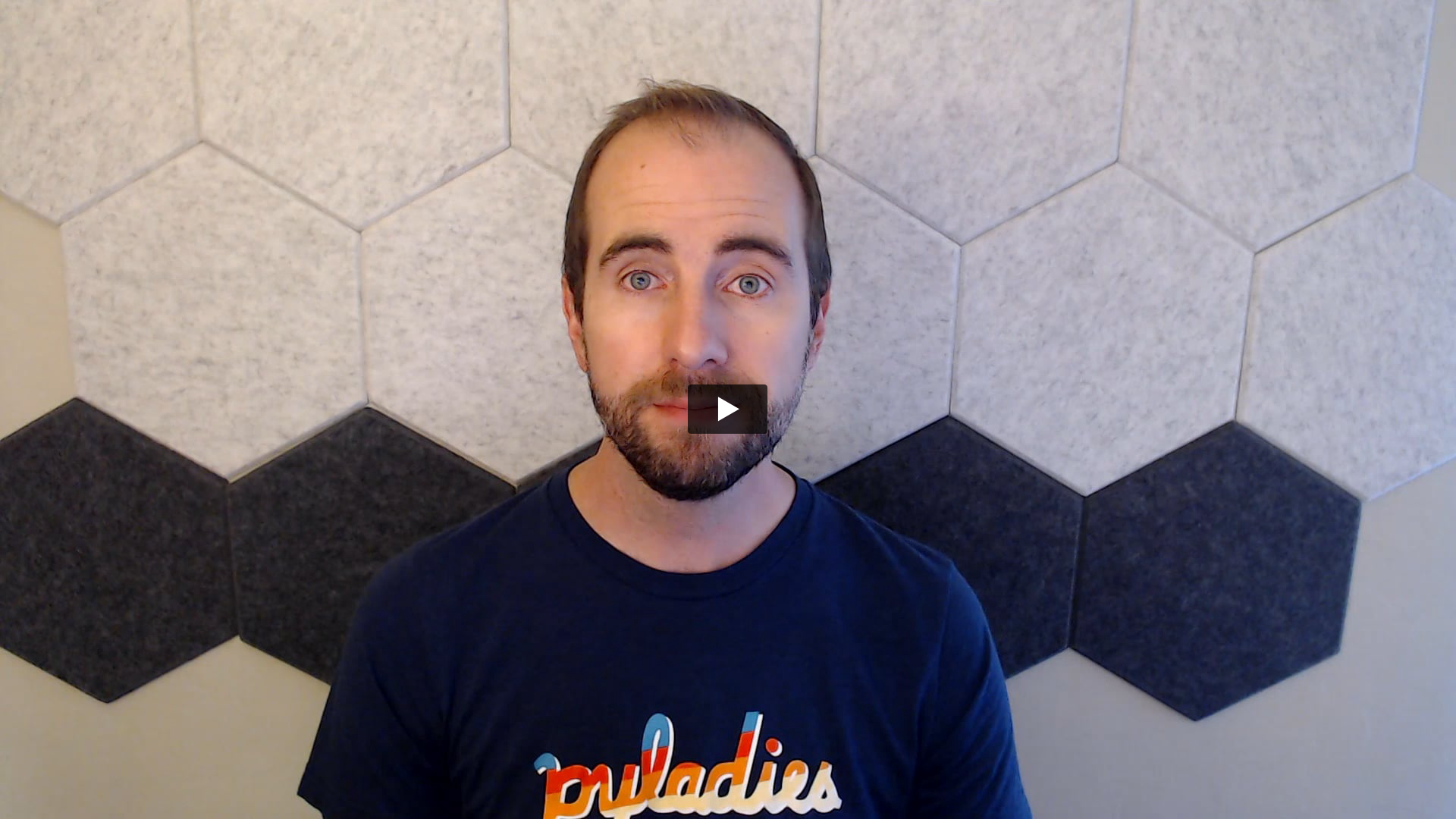
Table of contents
Reading a CSV file with csv.reader
The Python Standard Library has a csv
module, which has a reader
function within it:
>>> importcsv>>> csv.reader<built-in function reader>
We can use the reader
function by passing it an iterable of lines.
This usually involves passing reader
a file object, because files are iterables in Python, and as we loop over them, we'll get back each line in our file (see reading a file line-by-line in Python).
Here we have a CSV file called penguins_small.csv
:
species,island,bill_length_mm,bill_depth_mm,flipper_length_mm,body_mass_g,sex
Adelie,Torgersen,39.1,18.7,181,3750,MALE
Adelie,Dream,39.5,16.7,178,3250,FEMALE
Adelie,Biscoe,39.6,17.7,186,3500,FEMALE
Chinstrap,Dream,46.5,17.9,192,3500,FEMALE
Gentoo,Biscoe,46.1,13.2,211,4500,FEMALE
Let's use Python's built-in open
function to [open our file for reading][reading from a file].
>>> penguins_file=open("penguins_small.csv")
Now we can call pass the file object we got back to csv.reader
:
>>> penguins_file=open("penguins_small.csv")>>> reader=csv.reader(penguins_file)
When we call csv.reader
we'll get back a reader
object:
>>> reader<_csv.reader object at 0x7fd34c861930>
We can loop over that reader
object to get the rows within it:
>>> forrowinreader:... print(row)...
When we loop over a csv.reader
object, the reader
will loop over the file object that we originally gave it and convert each line in our file to a list of strings:
>>> forrowinreader:... print(row)...['species', 'island', 'bill_length_mm', 'bill_depth_mm', 'flipper_length_mm', 'body_mass_g', 'sex']['Adelie', 'Torgersen', '39.1', '18.7', '181', '3750', 'MALE']['Adelie', 'Dream', '39.5', '16.7', '178', '3250', 'FEMALE']['Adelie', 'Biscoe', '39.6', '17.7', '186', '3500', 'FEMALE']['Chinstrap', 'Dream', '46.5', '17.9', '192', '3500', 'FEMALE']['Gentoo', 'Biscoe', '46.1', '13.2', '211', '4500', 'FEMALE']
Each list represents one row in our file, and each string in each list represents the data in one column in that row.
Skipping the header row in a CSV file
Note that csv.reader
doesn't know …