Max Pain is a theory that suggests that the price of an option contract will tend to move towards a certain strike price, known as the "max pain" strike price, before the option expires. The idea behind this theory is that the option strike price at which the most options will expire worthless is the strike price that causes the most "pain" to option holders.
You can calculate the max pain strike price for a particular stock using pandas and the following steps:
Get the option chain data for the stock in question. This data should include the strike price, expiration date, and open interest for each option contract.
Group the option contracts by expiration date and strike price, and calculate the total open interest for each strike price.
For each expiration date, find the strike price with the highest total open interest. This is the max pain strike price for that expiration date.
Plot the max pain strike prices for each expiration date to visualize the max pain theory.
In this post, we will get the data from Yahoo finance using yfinance Python package.
Let us do an example first. Let us download the option data for expiry 2023-02-03 for APPLE stock.
importyfinanceasyfimportpandasaspd# Get the options data for a stockstock=yf.Ticker("AAPL")options_chain=stock.option_chain(date='2023-02-03')
options_chain contains options_chain.calls and options_chain.puts method.
pd.DataFrame(options_chain.calls).head(1)
contractSymbol | lastTradeDate | strike | lastPrice | bid | ask | change | percentChange | volume | openInterest | impliedVolatility | inTheMoney | contractSize | currency | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | AAPL230203C00060000 | 2023-01-06 16:03:12+00:00 | 60.0 | 67.72 | 76.7 | 79.15 | 0.0 | 0.0 | 6.0 | 6 | 1.742189 | True | REGULAR | USD |
There is stock.options which contains all the expirationDates.
stock.options
('2023-01-27', '2023-02-03', '2023-02-10', '2023-02-17', '2023-02-24', '2023-03-03', '2023-03-17', '2023-04-21', '2023-05-19', '2023-06-16', '2023-07-21', '2023-08-18', '2023-09-15', '2023-10-20', '2023-12-15', '2024-01-19', '2024-03-15', '2024-06-21', '2025-01-17', '2025-06-20')
Let us try to find the max pain for TSLA options.
importyfinanceasyfimportpandasaspdstock_ticker="TSLA"# Get the list of all available dates for the option chain datadates=yf.Ticker(stock_ticker).options# Initialize an empty DataFrame to store the option chain dataall_data=pd.DataFrame()# Loop through all available datesfordateindates:# Get the option chain data for the specific dateoption_chain=yf.Ticker(stock_ticker).option_chain(date=date)# convert the option chain data to a pandas dataframedf=option_chain.calls.append(option_chain.puts)# Extract the expiration date from the contractSymbol columndf['Expiry']=df['contractSymbol'].str[4:10]# Append the option chain data for the specific date to the all_data DataFrameall_data=all_data.append(df)# Group option contracts by expiration date and strike pricegrouped=all_data.groupby(['Expiry','strike'])['openInterest'].sum()# Find the max pain strike price for each expiration datemax_pain=grouped.groupby(level=0).idxmax()max_pain=max_pain.apply(lambdax:x[1])# Plot max pain strike pricesmax_pain.plot()
<AxesSubplot:xlabel='Expiry'>
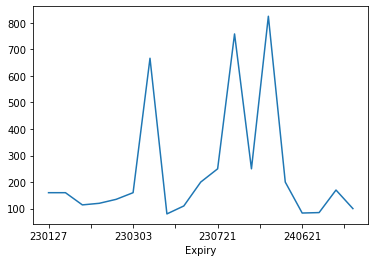
max_pain
Expiry 230127 160.00 230203 160.00 230210 114.00 230217 120.00 230224 135.00 230303 160.00 230317 666.67 230421 80.00 230519 110.00 230616 200.00 230721 250.00 230915 758.33 231215 250.00 240119 825.00 240315 200.00 240621 83.33 240920 85.00 250117 170.00 250620 100.00 Name: openInterest, dtype: float64